Many assembly tutorials and books doesn't coverhow to write a simple assembly program on the Mac OS X.Here are some baby steps that can help people whoare also interested in assembly to get startedeasier.
Mach-O file format
Devices and Mac OS X version. VLC media player requires Mac OS X 10.7.5 or later. It runs on any Mac with a 64-bit Intel processor or an Apple Silicon chip. Previous devices are supported by older releases. Note that the first generation of Intel-based Macs equipped with Core Solo or Core Duo processors is no longer supported. Handgun Hoedown is the local multiplayer Western game for having a hoedown with handguns! Get to a gun as quickly as possible and shoot your fellow cowboy right between the eyes - if you haven't got a revolver, run for your life or punch one right out of their hands! The first cowboy to three kills wins! By default, your iPad shows an extension of your Mac desktop. You can move windows to it and use it like any other display. To mirror your Mac display so that both screens show the same content, return to the Display menu or AirPlay menu, which shows a blue iPad icon while using Sidecar. Choose the option to mirror your display. The Military Armament Corporation Model 10, officially abbreviated as 'M10' or 'M-10', and more commonly known as the MAC-10, is a compact, blowback operated machine pistol/submachine gun that was developed by Gordon B. It is chambered in either.45 ACP or 9mm.A two-stage suppressor by Sionics was designed for the MAC-10, which not only abates the noise created, but.
To get started on writing OSX assembly, you need tounderstand OSX executable file format – the Mach-Ofile format. It's similar to ELF, but insteadof sections of data, bss, and text, it has segments thatcontains sections.
Make sure your version of tar supports all 4 different compressed file formats (.lz,.gz,.xz,.bz2), but since the standard Mac version of tar does that for me, it'll probably work for you too. On 2017-09-27, I failed to build GCC 7.2.0 on macOS High Sierra 10.13 (using XCode 9 for the bootstrap compiler) using the same script as worked on.
A common assembly in Linux like
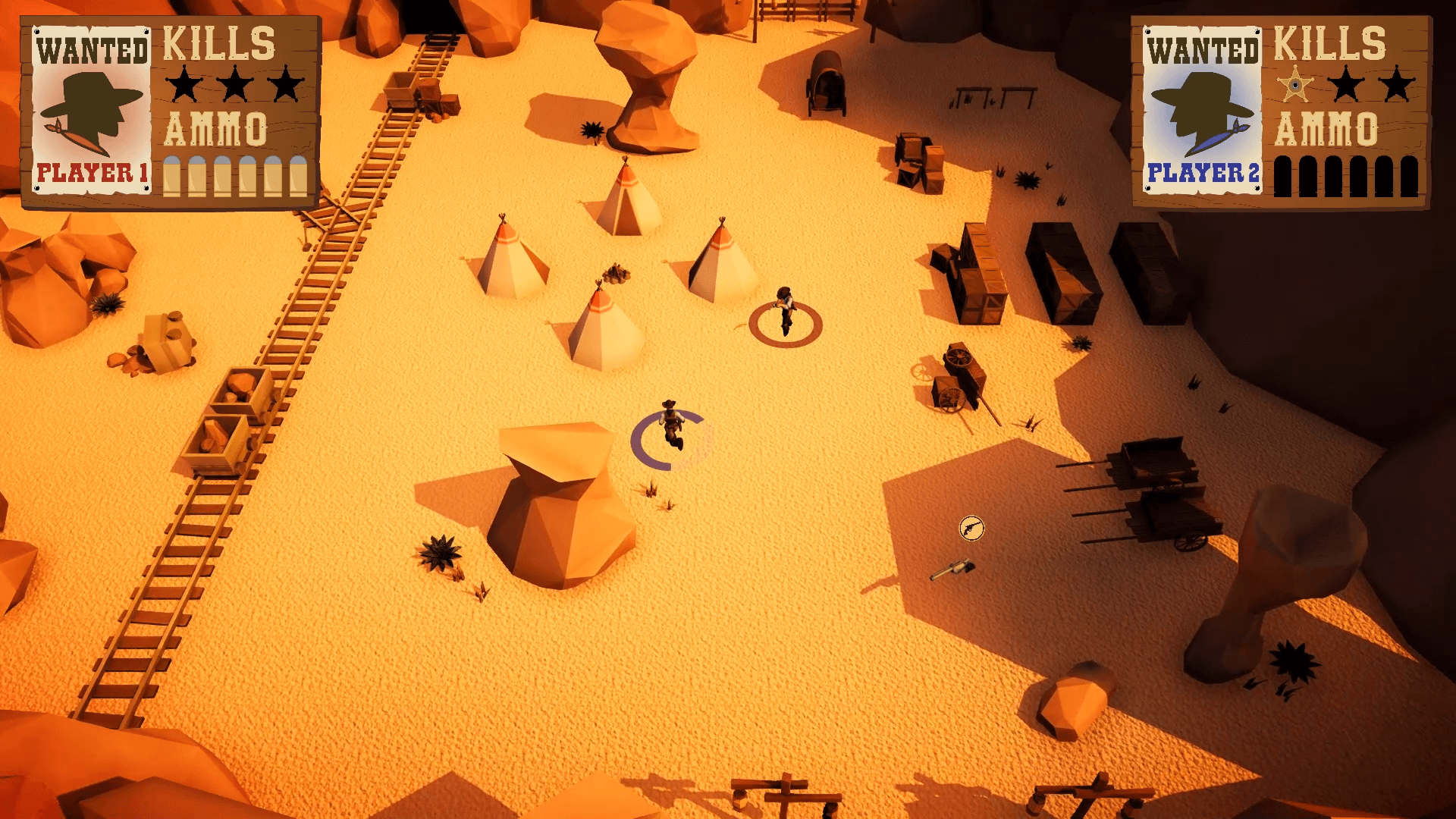
would translate into this in Mach-O
Mach-O is pretty flexible. You can embed acstring
section in your __TEXT
segment insteadof putting it in __DATA,__data
. Actually this isthe default behavior that compiler does on your Mac.
Hello Assembly
Now we know how to translate common linux assemblyto mac, let's write a basic program – do a system callwith an exit code.
Mac Os Mojave
On x86 you do a system call by int x80
instruction. On64 bit machine, you do this by syscall
. Here's the samplecode:
you can compile the code by the following commands:
To perform a system call, you put the system call number in%eax
, and put the actual exit code to %ebx
. The systemcall number can be found in /usr/include/sys/syscall.h
.
The system call number need to add an offset 0x2000000
, becauseOSX has 4 different class of system calls. You can find the referencehere XNU syscall.
System call by using wrapper functions
If you're like me that had no assembly background, you mightfeel that syscall
is alien to you. In C, we usually usewrapper functions to perform the call:
Now we call a libc
function instead of performing a systemcall. To do this we need to link to libc by passing -lc
to linker ld
. There are several things you need to doto make a function call.
Call frame
We need to prepare the stack before we call a function. Elseyou would probably get a segmentation fault.The values in %rsp
and %rbp
is used to preserve frame information.To maintain the stack, you first push the base register %rbp
onto the stack by pushq %rbp
;then you copy the stack register %rsp
to the base register.
If you have local variables, you subtract %rsp
for space.Remember, stack grows down and heap grows up.When releasing the frame, you add the space back to %rsp
.
A live cycle of a function would look like this:
The stack size can be set at link time. On OSX, below are theexample parameters you can pass to ld
to set the stack size:
When setting the stack size, you also have to set the stack address.On the System V Application Binary Interface it says
Although the AMD64 architecture uses 64-bit pointers, implementationsare only required to handle 48-bit addresses. Therefore, conforming processes may onlyuse addresses from 0x00000000 00000000
to 0x00007fff ffffffff
I don't know a good answer of how to chose a good stack address.I just copy whatever a normal code produces.
Parameters passing
The rules for parameter passing can be found in System VApplication Binary Interface:
- If the class is MEMORY, pass the argument on the stack.If the size of an object is larger than four eight bytes, orit contains unaligned fields, it has class MEMORY.
- If the class is INTEGER, the next available register of the sequence
%rdi
,%rsi
,%rdx
,%rcx
,%r8
and%r9
is used. - If the class is SSE, the next available vector register is used, the registersare taken in the order from
%xmm0
to%xmm7
.
The exit()
function only need one integer parameter, therefore we putthe exit code in %edi
. Since the parameter is type int
, we use 32 bitvariance of register %rdi
and the instruction is movl
(mov long) insteadof movq
(mov quad).
Hello world
Now we know the basics of how to performa system call, and how to call a function.Let's write a hello world program.
The global variable str
can only be accessed through GOT(Global Offset Table). And the GOT needs to be access fromthe instruction pointer %rip
. For more curious you canread Mach-O Programming Topics: x86-64 Code Model.
The register used for syscall
parameters are a littlebit different than the normal function call.It uses %rdi
, %rsi
, %rdx
, %r10
, %r8
and %r9
.You cannot pass more than 6 parameters in syscall
, norcan you put the parameters on the stack.
Hello world using printf
Now you know the basics of assembly. A hello worldexample using printf should be trivial to read:
Conclusion
The 64 bit assembly looks more vague than the tutorialswritten in X86 assembly. Once you know these basic differences,it's easy for you to learn assembly in depth on your own,even if the material is designed for x86. I highly recommendthe book 'Programming from the ground up'. It is well writtenfor self study purpose.
References
- OS X Assembler Reference Assembler Directives
- Book: Programming from the ground up.
Handgun Hoedown Mac Os Pro
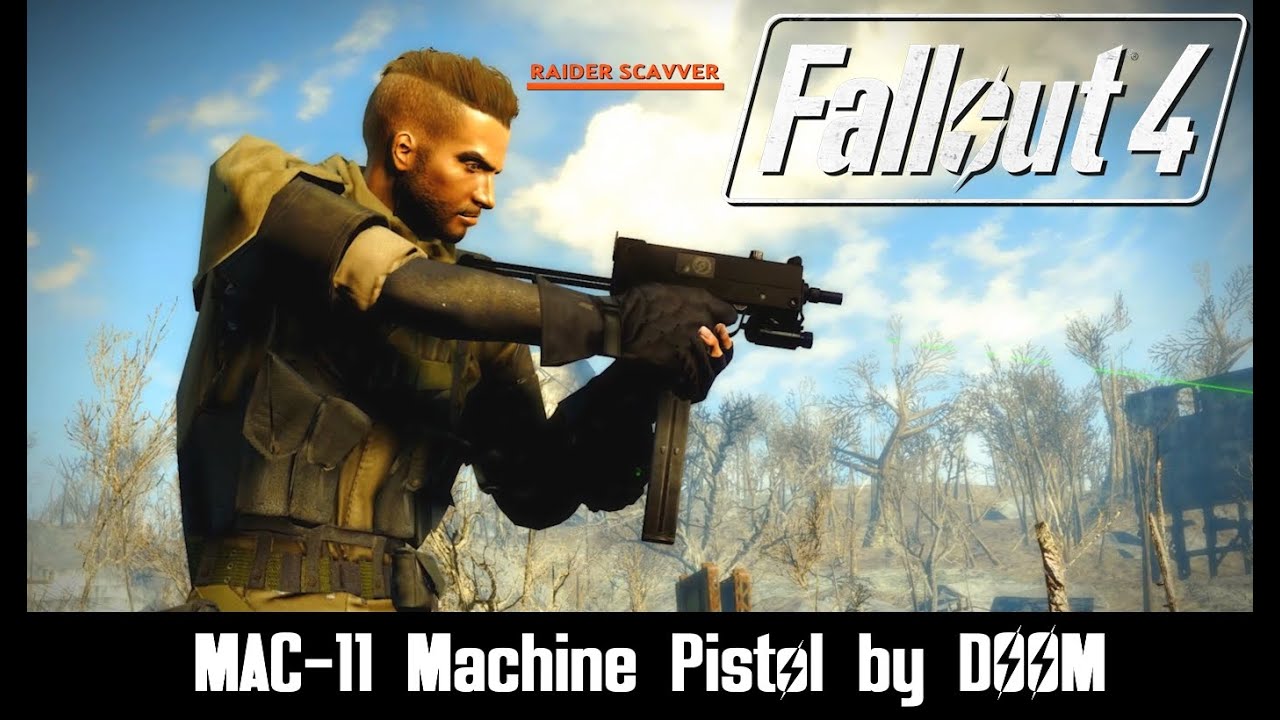
would translate into this in Mach-O
Mach-O is pretty flexible. You can embed acstring
section in your __TEXT
segment insteadof putting it in __DATA,__data
. Actually this isthe default behavior that compiler does on your Mac.
Hello Assembly
Now we know how to translate common linux assemblyto mac, let's write a basic program – do a system callwith an exit code.
Mac Os Mojave
On x86 you do a system call by int x80
instruction. On64 bit machine, you do this by syscall
. Here's the samplecode:
you can compile the code by the following commands:
To perform a system call, you put the system call number in%eax
, and put the actual exit code to %ebx
. The systemcall number can be found in /usr/include/sys/syscall.h
.
The system call number need to add an offset 0x2000000
, becauseOSX has 4 different class of system calls. You can find the referencehere XNU syscall.
System call by using wrapper functions
If you're like me that had no assembly background, you mightfeel that syscall
is alien to you. In C, we usually usewrapper functions to perform the call:
Now we call a libc
function instead of performing a systemcall. To do this we need to link to libc by passing -lc
to linker ld
. There are several things you need to doto make a function call.
Call frame
We need to prepare the stack before we call a function. Elseyou would probably get a segmentation fault.The values in %rsp
and %rbp
is used to preserve frame information.To maintain the stack, you first push the base register %rbp
onto the stack by pushq %rbp
;then you copy the stack register %rsp
to the base register.
If you have local variables, you subtract %rsp
for space.Remember, stack grows down and heap grows up.When releasing the frame, you add the space back to %rsp
.
A live cycle of a function would look like this:
The stack size can be set at link time. On OSX, below are theexample parameters you can pass to ld
to set the stack size:
When setting the stack size, you also have to set the stack address.On the System V Application Binary Interface it says
Although the AMD64 architecture uses 64-bit pointers, implementationsare only required to handle 48-bit addresses. Therefore, conforming processes may onlyuse addresses from 0x00000000 00000000
to 0x00007fff ffffffff
I don't know a good answer of how to chose a good stack address.I just copy whatever a normal code produces.
Parameters passing
The rules for parameter passing can be found in System VApplication Binary Interface:
- If the class is MEMORY, pass the argument on the stack.If the size of an object is larger than four eight bytes, orit contains unaligned fields, it has class MEMORY.
- If the class is INTEGER, the next available register of the sequence
%rdi
,%rsi
,%rdx
,%rcx
,%r8
and%r9
is used. - If the class is SSE, the next available vector register is used, the registersare taken in the order from
%xmm0
to%xmm7
.
The exit()
function only need one integer parameter, therefore we putthe exit code in %edi
. Since the parameter is type int
, we use 32 bitvariance of register %rdi
and the instruction is movl
(mov long) insteadof movq
(mov quad).
Hello world
Now we know the basics of how to performa system call, and how to call a function.Let's write a hello world program.
The global variable str
can only be accessed through GOT(Global Offset Table). And the GOT needs to be access fromthe instruction pointer %rip
. For more curious you canread Mach-O Programming Topics: x86-64 Code Model.
The register used for syscall
parameters are a littlebit different than the normal function call.It uses %rdi
, %rsi
, %rdx
, %r10
, %r8
and %r9
.You cannot pass more than 6 parameters in syscall
, norcan you put the parameters on the stack.
Hello world using printf
Now you know the basics of assembly. A hello worldexample using printf should be trivial to read:
Conclusion
The 64 bit assembly looks more vague than the tutorialswritten in X86 assembly. Once you know these basic differences,it's easy for you to learn assembly in depth on your own,even if the material is designed for x86. I highly recommendthe book 'Programming from the ground up'. It is well writtenfor self study purpose.
References
- OS X Assembler Reference Assembler Directives
- Book: Programming from the ground up.
Handgun Hoedown Mac Os Pro
Howard's Pawn and Jewelry handles nearly 10,000 firearms transactions in a single year.
While this does include pawn business where the gun was pawned and then picked up, it's a
substantial number. It shows the experience we have in handling guns, both old and new.
Our typical gun-buying customer is a working-class male between 40 and 60 years old.
But we serve a far greater group of gun enthusiasts than that demographic. Why? Because we carry such a wide range of firearms for all types of gun enthusiasts. If you're interested in buying or trading your weapon and you are not looking to shop the big box stores, we are your go-to for everything you need.
When people think of a pawn
business, they usually expect
all used goods.
While we have a large selection of used guns to choose from, you might be surprised
to know a large piece of our firearms sales consists of new guns. Our new gun sales
have grown significantly in recent years. We take trade-ins for new guns, we can
special order something you might not be able to find anywhere else locally, and we
price everything fairly and competitively.
The brands we carry include:
- Benelli
- Beretta
- Browning
- Colt
- Ruger
- Smith and Wesson
- Glock
- Springfield Armory
- Taurus
- And many more…
The firearms and accessories we sell:
- Rifles
- Home defense weapons
- Shotguns
- Pistols
- Silencers
- Scopes
- Ammunition
- Magazines
- Optics
- Lower receivers
- Holsters
In 2015 we also started selling class three firearms merchandise, including silencers,
short-barreled rifles, and fully automatic weapons (post 1986 restriction). Though no one but
the military can get new class three weapons manufactured after 1986, we get a wide
variety through sales and trade-ins. Rest assured, when a collector or trader brings in
their guns or accessories, we require the proper paperwork before we move forward.
Another huge benefit of dealing with Howard's Pawn and Jewelry for
your gun business – you can skip the trip to the sheriff's department.
We have a streamlined process to handle fingerprints, photos, and the
paperwork required by the state for firearms purchases (only needed for the purchase of silencers and short barreled rifles). We're a one-stop-shop for all of your gun needs.
One of the bonuses of buying and selling guns with Howard's friendly,
experienced team is the firearms trade-in deal. We not only pawn and sell
firearms, but we also offer tremendous trade-ins. Our customers
consistently tell us our prices are far fairer than other pawn shops in the
middle Georgia area. We are very aggressive with our trade-in program and
pricing, so you know you're getting the best deal when you're ready to
upgrade your firearm.
You'll find more than guns
when you shop at Howard's.
Handgun Hoedown Mac Os Catalina
We always have a comprehensive collection of safety products available.
Whether you desire preventative measures for your home or items to carry with
you when you're camping, hunting, or simply driving around, we've got what you
need. If you're looking for top-of-the-line flashlights, pepper spray, or knives of
all kinds, we've got you covered.
We want you to be
satisfied with your purchase.
Mac Os Catalina
Just because we're a pawn shop doesn't mean we don't guarantee what we
sell. We offer a three-week trial period for any used gun you purchase from our
store. If, for any reason, the gun you purchased does not function as it
should, we will fix it at our own expense, or if it's not fixable, we will give you
a full credit toward a swap. This is not a rental or trade-in program – we
won't replace it because you decide you don't like it. But we will make good
on our promise to sell firearms that work as they should.
Are you in the market for a used or new firearm? Before you go to a big box
store or an overpriced gun shop, check out the selection at Howard's Pawn
and Jewelry. We think you'll be pleasantly surprised at the quality, selection,
and prices. Our team can't wait to help you find exactly what you need at a
price that works for your budget.